Easy Swag
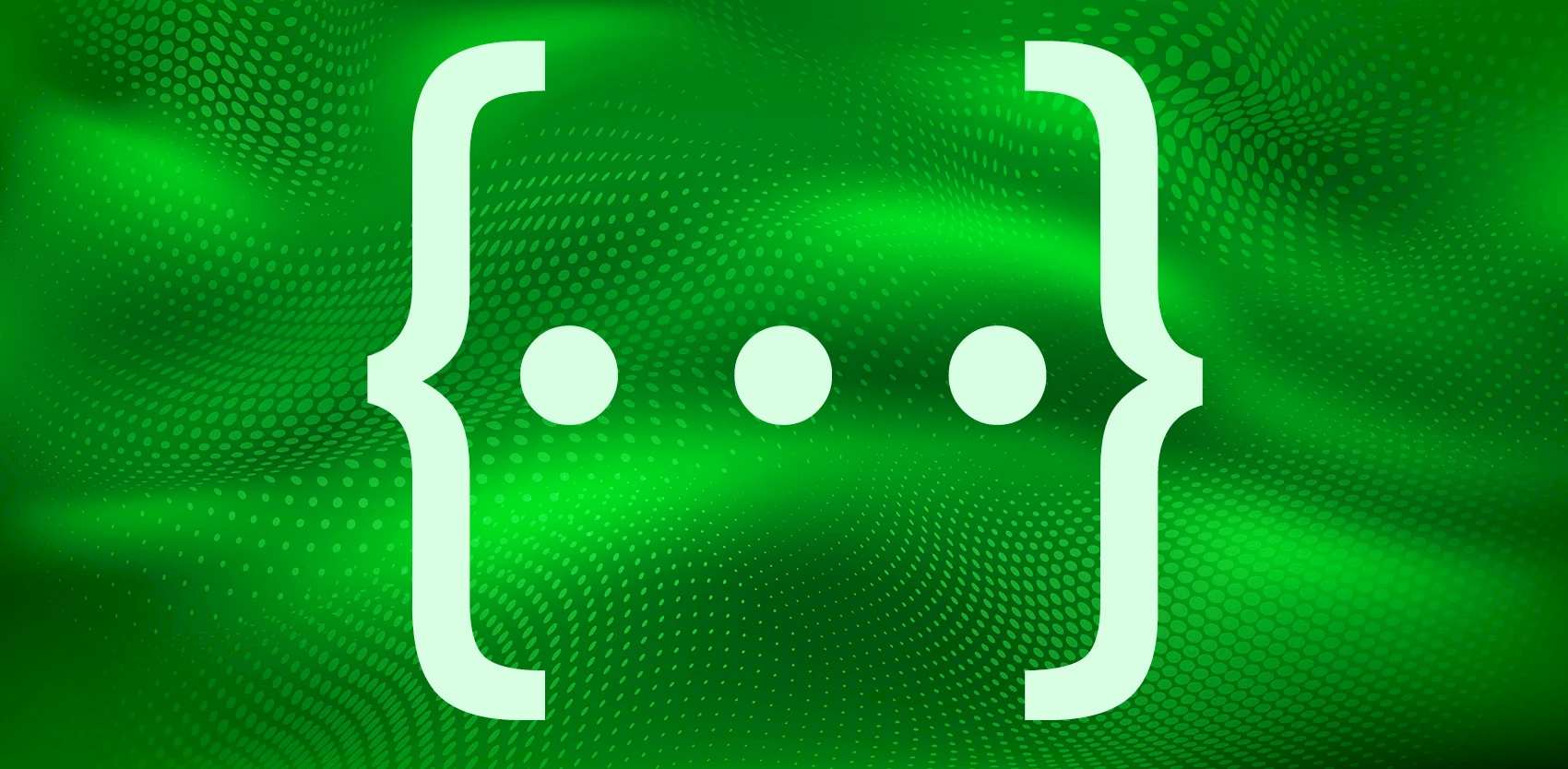
The swagger / OpenApi documentation standard provides an easy way to help developers use your REST API. Python offers multiple modules to help you generate the swagger doc, and though they already make your life easier, there is always room to make life even more so.
I worked on the back-end of a web project that used the popular Flask web server and Marshmallow schema marshalling modules. Multiple Python modules already help generate the Swagger documentation, but it is always possible to make it even easier. That’s why I wrote easy swag.
Description
The easy swag module is built on the “flask_apispec” module and the “marshmallow” module. It contains two functions and a decorator:
set_error_schema()
: sets the Marshmallow schema to be used as the error report for all REST API.register_with_swagger_docs()
: registers all swagger/OpenApi docs that have been declared with the Flask app.swag()
: the decorator that takes input and output schemas, the documentation string, tag and returned HTTP status code. It generates the proper doc.
Example
Here are a few excerpts from a demonstration Flask app.
First, register the Marshmallow schema used to report errors in the REST API. In this example, we declared an ExpectedError
schema class.
Python
# Set the error schema used in function
# and documented in swagger doc.
set_error_schema(schemas.ExpectedError, ‘400, 403’)
Second, decorate the Flask route with the easy-swag decorator. For example, here is the /dogs route. It uses the @swag
decorator, passing it the output schema, the documentation and tag. Because the REST end-point does not take input data and uses the default 200 status code for success, it does not need to be specified. As you can see, a single-line decorator is all it takes.
Python
@app.route(‘/dogs’, methods=[‘GET’])
@swag(output=schemas.Dogs, doc=‘Retrieve all dogs’, tag=‘dogs’)
def dogs_get_handler():
# ... your implementation code would be here ...
The decorator supports these elements:
input
: the input Marshmallow schema, describing the received JSON.output
: the output Marshmallow schema, describing the returned JSON.doc
: the documentation describing the REST end-point.tag
: the tag used to group related end-points together.success
: the status code returned in case of success.
Finally, register all documentation that has been declared. A simple call to a function is all that’s needed. It takes the Flask app and the name of your REST API. Optionally, it can also take the version of your REST API and the URL under which to serve the OpenApi documentation.
Python
register_with_swagger_docs(app, title = ‘web-example-app’)
Availability
You can find the easy-swag module in a demonstration Flask app that I posted on GitHub as part of my resto repo.
The easy-swag module is contained in a single file in src/flask-app/easy_swag.py.