The React ecosystem
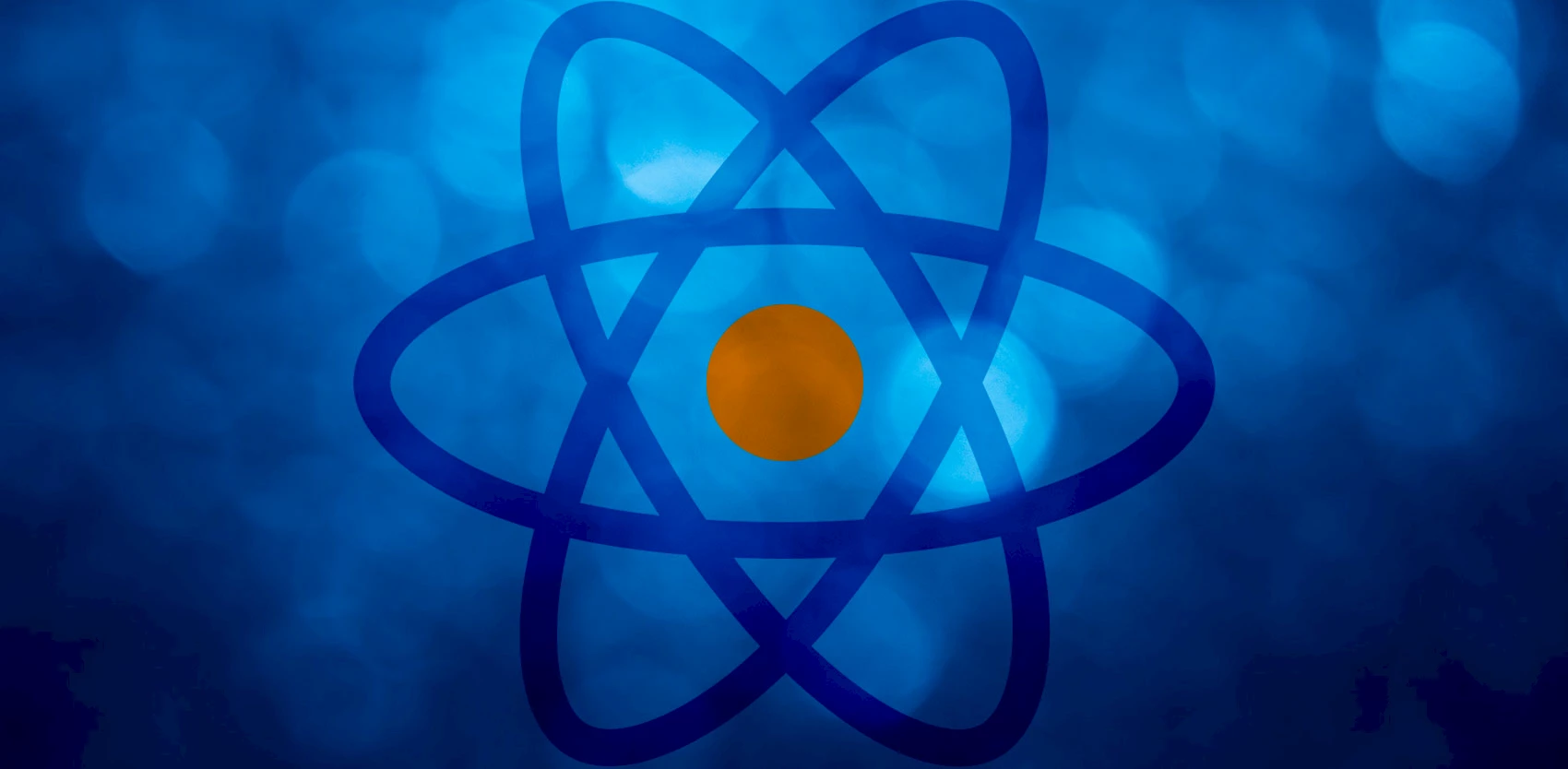
Jeff Sanchez, a consultant at Spiria Boston, explains how React, combined with Redux and ES6/ES7 in a Node environment, provides an intuitive, flexible and undemanding environment for quick-and-dirty Web application development. This article, originally written in 2016, has been updated and augmented.
It does seem like there’s no end to the new-kid-on-the-block JavaScript frameworks and libraries aimed at making our web development lives better. Framework fatigue has been a topic of discussion for years now with JavaScript developers. Many have tried building complex applications with Dojo, YUI, ExtJS, jQuery UI, Backbone, Ember, Cappuccino, SproutCore, GWT—oh man remember GWT? —Angular 1, Angular 2, Sencha, jQuery Mobile, Knockout, Meteor, Ampersand, Flight, Mithril, Polymer, React, etc. it just seems to never end!
Enter React
But if you haven’t looked at React yet, you’re probably (justifiably) skeptical about investing time in learning yet another library or framework for web development. It would have to be really good—clearly better than similar solutions—to bother with, right? Well, after quickly building a complex React application in the healthcare space, we’re happy to report that it is. And it gets even better when you consider the rapidly expanding React ecosystem. Since React is just a library (as opposed to some opinionated, potentially rigid framework), it’s easy to just dip your toes into the React world without much commitment. Also, it’s one-way data flow paradigm (originally called Flux, but now has Redux as its de facto standard it seems) is fairly easy to reason about and add into the mix of your application.
So what about some other popular choices one often hears in the same context as React? There’s Angular 1.x, which we did consider at the time, but had problems with the difficulty of debugging its two-way data flow in more complex situations, its breaking changes in the most recent Angular version, and its more opinionated way of doing things, being a more heavyweight framework. (Angular 2 would’ve been a better choice but wasn’t really ready at the time.) Ember is another popular choice (or used to be, anyway), but it’s a bit too opinionated and a steep learning curve for our tastes, at least compared to React. Plus it uses the less intuitive two-way data binding approach as well.
Flux, an approach to managing data flow in one direction, was another consideration in using React. As mentioned, the two way data binding approach of Angular and Ember has caused performance issues along with adding unnecessary complexity to large applications.
Flux “eschews MVC in favor of a unidirectional data flow” as Facebook says, which basically just means it’s easier to reason about data flowing through your application, especially when using Redux (a particular variant of Flux).
More specifically, the unidirectional data flow pattern of Flux allows for a simpler code base that’s less buggy. It also works well with React and the virtual DOM aspect of React. For more information on Flux, click here.
Our Toolkit
Here is the list of tools that we have used in projects along with React and Flux:
In addition to deciding on React, we also decided on using ECMAScript 6 (“ES6”), the 2015 version of JavaScript. There are many new language features/constructs that make React development much nicer (e.g., classes, template strings, arrow functions, spread operators) with ES6, and we can easily use Babel to transform our ES6 code into ES5 code which is supported by almost all browsers (that we care about, anyway!). We also decided to use Webpack for source code bundling, minifying, SCSS transformation, Babel management, etc. as it’s very good at all of these, and the industry seems to be converging on Webpack.
Advantages of React
One of the nicest things we noticed when we first started to experiment with React is that it's simple and flexible - we really could use as little of it as we'd like in a project, which made it very easy to try small React experiments in an application that mostly used some other UI or MVC related library/framework. As they say in the documentation, React is basically just the V in MVC—you’re not required to manage models, controllers, services, etc. And it’s flexible from the point of view of JavaScript versions as well—pretty much equally straightforward to create React components in ES5, ES6 (or even ES7 if you’re feeling particularly bleeding edge … don’t worry, Babel will make it all work out fine).
Another great, differentiating aspect of React for building modern UIs is the ease with which one can transform a mockup/static HMTL for a particular page gradually into a fully functioning React-ified page, complete with persistence of the page’s state, communication with external data sources, etc. We found it to be a pretty intuitive process:
- Start with the mockup page UI;
- Divide the page up into visual components (by the way, we’ve found Pattern Lab to be quite useful in this step in managing what’s basically a “living style document”);
- Decide what the state of the components should be (and which components make the most sense to store the state);
- Add our state-management code (we have various options here—more to follow on that topic), and
- Finally, wire up behaviors (basically actions involved in data flow/application state change).
But a less obvious, though probably more important React benefit (at least, for larger-scale production-ready web applications) is its virtual DOM + associated efficient DOM diff-ing algorithms. Why would I care about such things, you say? Because it makes a big difference performance-wise when you’ve got a page with a large number of components that potentially need to be re-rendered. React efficiently figures out just what portion of the DOM needs rerendering (via its virtual DOM representation of the real DOM) and then only renders that portion. For a small application it doesn’t matter, but if you’ve got grids with a hundred rows and fifty columns with editable components in the grid, etc. that’s when you really start to see React shine (especially when compared with frameworks like Angular 1.x).
Because React has the full support of Facebook (and oh by the way, Instagram is implemented in React, so evidently React is “ready for prime time”), you also get a large, enthusiastic developer base moving it forward and providing additional support, libraries + ideas for tackling most every web application pattern and problem you’re likely to come across. This was key for us to committing to a new approach to web development. One particularly attractive solution we found in the React community to a generic problem of any application developer is that of managing application state.
The Case for Redux
If you’ve looked at React, you’ve probably already seen some mention of Flux, which is their suggested “pattern of unidirectional data flow.” (Specifically, data flows from views, to actions, to a dispatcher, to stores, and then back to views.) Flux has various implementations, and helps organize the application state in stores associated with different components in the application. Redux in a sense goes one step further than Flux, and simply stores the entire application state in one store as a tree data structure. Initially it may sound like a dubious approach for a complex app, but it turns out that it is pretty wonderfully simple and elegant. Furthermore, the entire application state is one big immutable tree structure (i.e., any change in the application state produces a whole new version of that tree). It may not be immediately obvious, but this greatly simplifies things like undo/redo functionality, playback/time travel debugging, and general ease of debugging of the entire application.
Potential Pitfalls
As for problem areas/potential pitfalls in React—so far, we haven’t really encountered significant ones for our development purposes, but these may be more or less of a concern to you:
- JSX (the XML-ish JavaScript extension syntax for React) can take a bit of getting used to, and some people find the context switching between JSX code and JavaScript a bit jarring to read.
- The available component library, though pretty decent and growing (see React.rocks or react-components.com for some good options), is not as well developed as, say, Angular, so you may find yourself doing a bit more “roll your own” components. We’ve had to do this in a few places (e.g., for things like nested tabs, and nested modals) but we found the additional development efforts to be pretty easy.
- Adopts a unidirectional data flow basically by default, so if you’re really looking for two-way data flow, something like Angular may make more sense.
Update for 2017
Since this article was first written, there have been various improvements to the React/Redux ecosystem which further supports our view that React is the way to go for complex web application development. Some of those are:
- Easier setup for new projects with create-react-app (don’t have to worry about all the tooling install as it’s done for you), which is heavily documented and supported by Facebook community.
- Standardization on Redux these days for state management (as opposed to the earlier many versions of Flux a few years back), or for simpler projects (in terms of state management), Mobx (a simpler version of Redux basically).
- Changed licensing to MIT license to be more favorable around patents, after pressure from the community to improve the licensing situation. (Automattic’s high profile complaints about the React license may have been what finally caused Facebook to change this.)
- Adoption of libraries like Redux-Saga and generator functions in ES2017 (ES7), for easier-to-understand and manage code involving asynchronous calls (side effects).
- Introduction of yarn package manager to help address issues around versioning of npm libraries.
- Widespread adoption of Storybook for designing React components (a tool sort of like Patternlab mentioned earlier in this article).
- Solid testing libraries like Enzyme and Jest available now and widely used.
- More support in full-featured, nicely styled React component libraries from organizations like PrimeFaces.
- Surpassed 50,000 stars on Github, and continues to be more popular than Angular.
Conclusion
React, along with Redux and ES6/ES7 in a Node environment provide a more intuitive, flexible, less committing UI ecosystem for quickly developing modern single page web applications. It has a large and growing community, and is easy to use in small doses without much downside/commitment, or start from scratch on a complex application. I encourage you to explore the React ecosystem and see how quickly you can get up and running with something cool!
Further Reading
- A Cartoon Intro to Redux.
- Learning React.js: Getting Started and Concepts.
- My Road To Redux Slideshare.